Here I describe a few simple tricks to make your programs run better.
To help speed up your BBS Basic programs here are some tips. They are meant as simple substitution to your existing code and a guide to better coding in the future. These are not an exhaustive list and have been created from reading stuff and trial and error so there is no warranty here!
IF AND vs IF THEN IF
With IF AND statements all parts of the expression are evaluated. These can easily be replaced with IF THEN IF which do the same job but if any conditional test fails then the rest of the statement does not both to execute!
So this:
IF X%>0 AND Y%>0 THEN I%=1
Is the same as:
IF X%>0 THEN IF Y%>0 THEN I%=1
But in execution if X%<>0 then the rest of the statement is not executed, a nice return.
In practice this can be a massive difference in performance. Here is a simple program that can test this:
10 MODE 7
20 PRINT "BBC BASIC PERFORMANCE TESTS"
30 PRINT "==========================="
40 REM GET MACHINE TYPE
50 Q%=1000 : REM DEFAULT TO BBC
60 IF TOP> 300000000 THEN Q%=100000: PRINT "PC MODE" ELSE Q%=1000: PRINT "BBC MODE"
70
80 PRINT "IF AND vs IF THEN IF"
90 Z%=0
100
110 PRINT "IF AND TEST"
120
130 TIME=0
140 T = TIME
150
160 FOR I%=1 TO Q%
170 FOR J%=1 TO 5
180 IF J%=1 AND J%>0 THEN Z%=1
190 IF J%=2 AND J%>0 THEN Z%=2
200 IF J%=3 AND J%>0 THEN Z%=3
210 IF J%=4 AND J%>0 THEN Z%=4
220 IF J%=5 AND J%>0 THEN Z%=5
230 NEXT
240 NEXT I%
250
260 PRINT "TIME:";(TIME-T%);" "
270
280 PRINT "IF THEN IF THEN TEST"
290
300 TIME=0
310 T = TIME
320
330 FOR I%=1 TO Q%
340 FOR J%=1 TO 5
350 IF J%=1 THEN IF J%>0 THEN Z%=1
360 IF J%=2 THEN IF J%>0 THEN Z%=2
370 IF J%=3 THEN IF J%>0 THEN Z%=3
380 IF J%=4 THEN IF J%>0 THEN Z%=4
390 IF J%=5 THEN IF J%>0 THEN Z%=5
400 NEXT
410 NEXT I%
420
430 PRINT "TIME:";(TIME-T%);" "
Here are the results running on the BBCBasic IDE on a Windows PC:
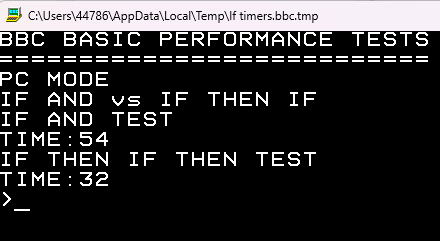
What is clear is that the THEN IF is massively quicker!
A quick note on the program. At the top of the listing there is a check against TOP, which returns the top of memory. It is a simple way to detect if you are running on the PC or a BBC. It sets the correct Q% value that is used to govern the number of loops to take during the tests.
Here is the same program running on a BBC:
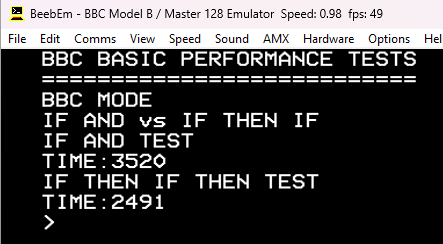
On the BeeB there is still a massive difference.
With lots of conditional tests in a loop it is a big upgrade.
FLOAT vs INTEGER, NEXT <VAR> and IF CONST/VAR
Use integer rather than floats.
For probably most programs you don’t need floats. If you are using variables with a range of say 1 to 10 then floats are pointless.
Omit the variable on NEXT statements
When in a FOR – NEXT loop you typically specify the variable in the NEXT. In fact this is not needed for program flow and it actually cost more in CPU cycles as the variable has to be interpreted:
FOR A%=1 TO 10:REM DO SOMETHING:NEXT A%
If slower then:
FOR A%=1 TO 10:REM DO SOMETHING:NEXT
Use variables rather then constants in conditionals
When testing a condition against a constant such as:
IF X%=10 THEN REM DO SOMETHING
It turns out to be slower than:
Z%=10
IF X%=Z% THEN REM DO SOMETHING
Looks odd but just like the NEXT the interpreter has to pass what 10 is rather then passing a reference to the variable value in memory. Of course in this case you should place Z%=10 somewhere at the top of the program and make use of this technique if you are using it more than a couple of times.
Here is a program to test this:
10 MODE 7
20 PRINT "BBC BASIC PERFORMANCE TESTS"
30 PRINT "==========================="
40 REM GET MACHINE TYPE
50 Q%=1000 : REM DEFAULT TO BBC
60 IF TOP> 300000000 THEN Q%=10000000: PRINT "PC MODE" ELSE Q%=5000: PRINT "BBC MODE"
70
80
90 PRINT "LOOP WITH FLOAT"
100 TIME=0
110 T = TIME
120 FOR X=1 TO Q%:NEXT X:
130 PRINT " TIME:";(TIME-T%)
140
150
160 PRINT "LOOP WITH INTEGER"
170 TIME=0
180 T = TIME
190 FOR X%=1 TO Q%:NEXT X%
200 PRINT " TIME:";(TIME-T%)
210
220
230 PRINT "LOOP WITH INTEGER WITHOUT NEXT <VAR>"
240 TIME=0
250 T = TIME
260 FOR X%=1 TO Q%:NEXT
270 PRINT " TIME:";(TIME-T%)
280
290
300 PRINT "CONDITIONAL BY CONSTANT"
310 TIME=0
320 T = TIME
330 FOR I%=1 TO Q%
340 IF I%=10 THEN O=O+1
350 NEXT I%
360 PRINT " TIME:";(TIME-T%)
370
380
390 PRINT "CONDITIONAL BY VARIABLE"
400 TIME=0
410 T = TIME
420 J%=10
430 FOR I%=1 TO Q%
440 IF I%=J% THEN O=O+1
450 NEXT I%
460 PRINT " TIME:";(TIME-T%)
470
480
490
Here is a break down of performance:
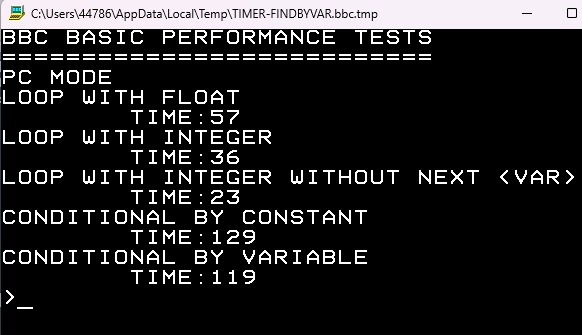
And on BeeB:
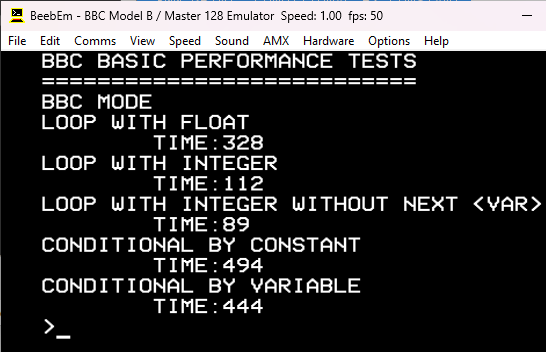
Float vs Int is amazing. Just a simple change of adding a % is great. The NEXT is a no brainer.
The check by const vs var is a smaller benefit but if you do this lots in your program then why not?
So that’s it for a bit but tune in next time for more program stuff…
Check it out on YouTube: